Sensitive Data Encryption and Decryption
Overview
When handling API requests, especially those involving sensitive data (such as card numbers, CVV, expiration dates, etc.), it's essential to ensure the security of the data. To protect this sensitive data, we use the RSA
encryption algorithm combined with the ECB
mode and OAEPPadding
padding scheme.
RSA Encryption
Encryption Algorithm
RSA/ECB/OAEPPadding
- RSA: An asymmetric encryption algorithm that uses a public key and a private key to encrypt and decrypt data.
- ECB (Electronic Codebook Mode): An encryption mode where each block is encrypted independently, suitable for encrypting plaintext blocks of the same length.
- OAEPPadding: A padding scheme in RSA that provides additional security and prevents certain types of attacks.
Encryption and Decryption Process
- Generate Key Pair:
- Generate an RSA public and private key. The public key is used for encrypting data, and the private key is used for decrypting data. Please refer to the previous document Generate Key Pair to generate your keys. Store your private key securely, and upload your public key to the CodePay platform.
- With RSA encryption, you only need to use CodePay's public key, so you don't need to generate your own keys. If you need to decrypt sensitive data returned by CodePay, you will need your private key. Currently, in business scenarios, sensitive data is not returned to developers, so this step can be temporarily ignored.
- Encrypt Sensitive Data:
- Encrypt sensitive data using the RSA public key. In our API usage scenario, when you request CodePay, encrypt the data using CodePay’s public key
GATEWAY_RSA_PUBLIC_KEY
. - During the encryption process, use ECB mode and the OAEPPadding padding scheme.
- Transmit Encrypted Data:
- Encode the encrypted byte array as a Base64 string to facilitate transmission over the network. The encrypted data will be transmitted through the API.
- Decrypt Data:
- The recipient decodes the Base64 string and decrypts the data using the RSA private key.
- During the decryption process, the same padding scheme (OAEPPadding) is used.
When you receive encrypted data in CodePay's response or Webhook notification message, use your private key APP_RSA_PRIVATE_KEY
to decrypt it in the same manner.
Important Notes
- Key Management: Ensure secure management of both public and private keys, and do not expose the private key to unauthorized personnel.
- Data Security: Ensure that data remains confidential before encryption and that no plaintext data is leaked during transmission.
- Compliance: Follow applicable regulations and standards (e.g., PCI-DSS) when handling sensitive data.
Frequently Asked Questions
- Why does RSA encryption produce different results each time when encrypting the same plaintext?
The different results in RSA encryption occur because of the use of random padding mechanisms. This is an important feature of RSA encryption that enhances security and prevents encryption mode attacks.
- Random Padding: Each time data is encrypted, the padding algorithm (e.g., OAEP) generates new random data, which is then encrypted along with the plaintext. Therefore, even if the plaintext is the same, the encryption result will differ due to different random padding.
- Prevention of Replay Attacks: Through random padding, RSA encryption avoids the same ciphertext being generated from repeated encryption operations, which is important for preventing certain attacks like replay attacks.
Therefore, as long as decryption ensures success, the encryption process can be considered correct.
References
Sample Code
Here is a sample code using the Java SDK that demonstrates how to use RSA for encryption. For the complete code, please refer to Java SDK:
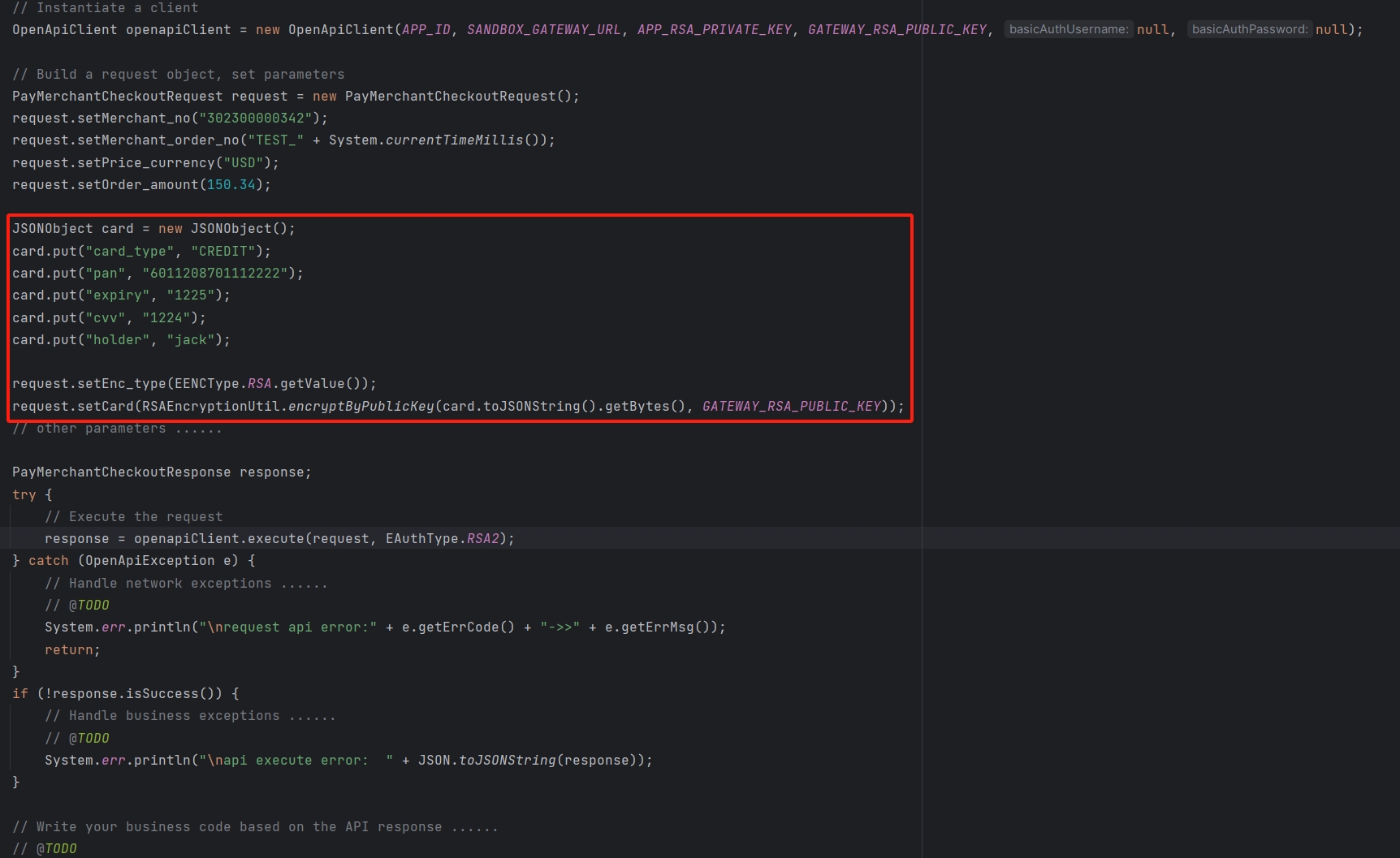
AES Encryption
AES (Advanced Encryption Standard) is a symmetric encryption algorithm widely used in data protection. Unlike RSA, AES uses the same key for both encryption and decryption, offering efficient and robust encryption capabilities.
Encryption Algorithm
AES/ECB/PKCS5Padding
AES: A symmetric encryption algorithm that uses a fixed key size (128 bits) to encrypt and decrypt data. AES supports multiple key lengths, including 128, 192, and 256 bits.
ECB (Electronic Codebook Mode): A commonly used encryption mode that splits the input data into fixed-size blocks (typically 128 bits) and encrypts each block independently. While ECB mode is simple in encryption operations, it is vulnerable to certain attacks (e.g., mode analysis attacks) and is not recommended for use in highly sensitive applications.
PKCS5Padding: A padding scheme that ensures the block length matches the algorithm's requirements. If the data block is not long enough, PKCS5Padding will pad it to the appropriate length. PKCS5Padding is suitable for symmetric encryption algorithms to ensure each encrypted block is complete.
Encryption and Decryption Process
Generate Key: Choose an appropriate key length (e.g., 128, 192, or 256 bits). The key generation process should ensure its security to prevent unauthorized access.
Encrypt Sensitive Data:
- Encrypt sensitive data using the selected key in AES. During encryption, use ECB mode to encrypt each data block independently and apply the PKCS5Padding padding scheme to ensure the data block's length is appropriate.
- Transmit Encrypted Data:
- Encode the encrypted data as a byte array (usually using Base64 encoding) to facilitate transmission over the network. The encoded encrypted data can be returned as part of the API response to the client.
- Decrypt Data:
- The recipient decodes the Base64-encoded data and then decrypts it using the same AES key and padding scheme (PKCS5Padding) to restore the original plaintext data.
Important Notes
- Key Management: Ensure secure storage and management of AES keys to avoid key leakage. A secure key management system should be used to protect the key.
- Padding Scheme: The PKCS5Padding padding scheme is used to fill any gaps in the data block's length, but in some applications, if there are specific length requirements, other padding methods (like PKCS7) may be preferred.
- Limitations of ECB Mode: While ECB mode is simple to use, it is vulnerable to certain attacks because it does not introduce any randomization. In high-security scenarios, other modes (such as CBC mode) are often recommended.
Frequently Asked Questions
Why is the ciphertext different every time with AES encryption? AES encryption, when using certain modes (like CBC), involves randomization. However, ECB mode does not have this feature, so if the ciphertext is the same every time, it could be due to issues with the padding scheme or other encryption parameters. Note that ECB mode always produces the same ciphertext for identical input data, which can lead to security issues, so it is generally advisable to avoid it in certain applications.
How can the security of AES encryption be improved? While AES itself is very secure, using ECB mode may lead to predictable encryption patterns. It is recommended to use more secure encryption modes (like CBC or GCM), along with appropriate randomization strategies (like an IV initialization vector), to enhance encryption security.
References
Sample Code
Here is a sample code using the Java SDK that demonstrates how to use AES for encryption. For the complete code, please refer to Java SDK:
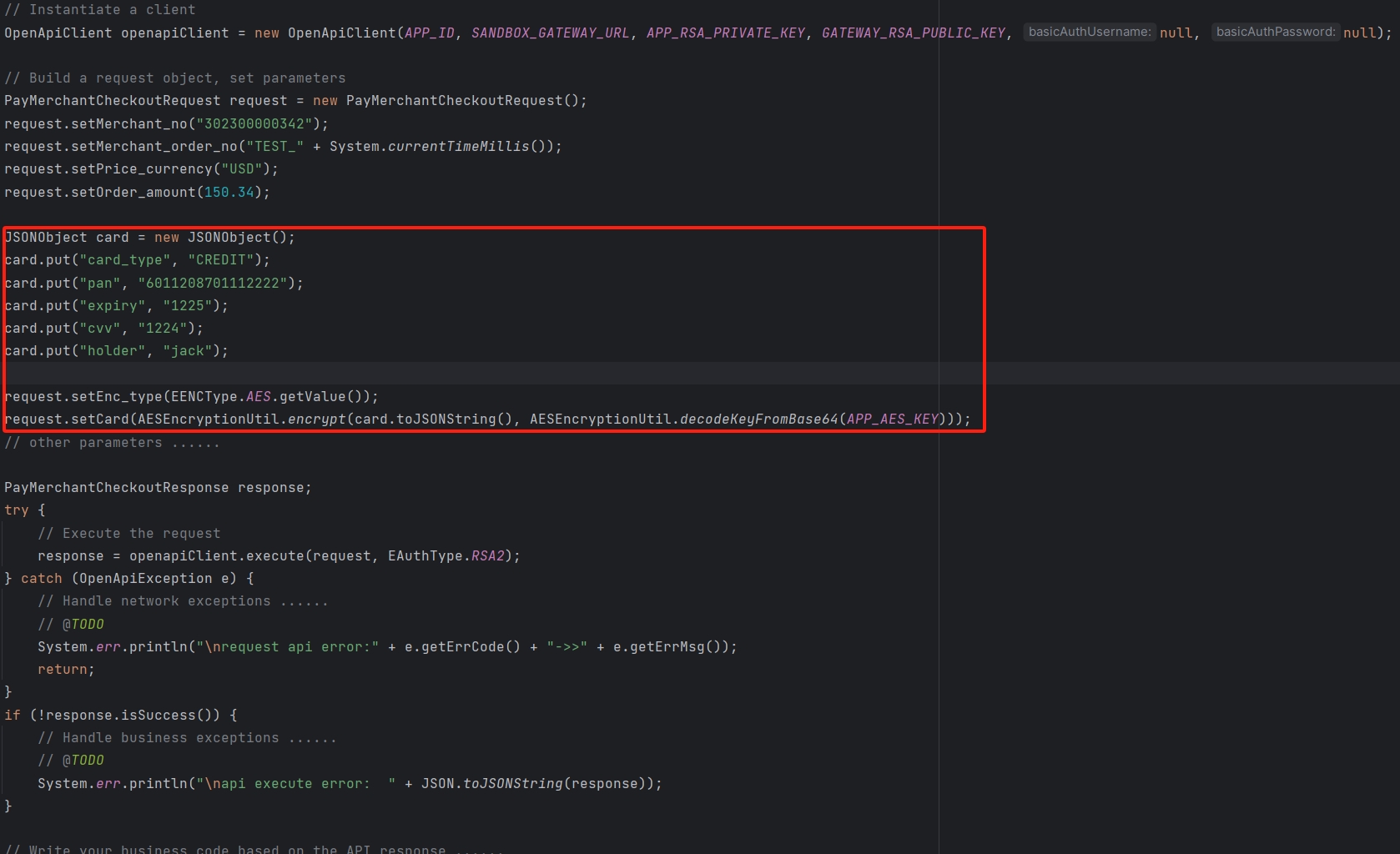